@sky_rain
CCU: Clock Controller Unit (CCU)
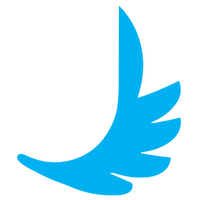
KunYi 发布的帖子
-
回复: F133 RTSCTS LINE
Okay, Maybe you can try the below code
#include <stdio.h> #include <fcntl.h> #include <errno.h> #include <termios.h> #include <unistd.h> #include <sys/ioctl.h> #include <linux/serial.h> #include <string.h> int main(int argc, char *argv[]) { // Open serial port ttyS1 const char *device = "/dev/ttyS1"; int fd = open(device, O_RDWR | O_NOCTTY); if (fd < 0) { perror("Error opening serial port"); return -1; } // Get the current serial port settings struct termios tty; if (tcgetattr(fd, &tty) < 0) { perror("Error getting port attributes"); close(fd); return -1; } // Configure the serial port settings // Clear all settings first tty.c_cflag = 0; tty.c_iflag = 0; // Enable receiver, ignore modem control lines tty.c_cflag |= CREAD | CLOCAL; // Enable hardware flow control (RTS/CTS) tty.c_cflag |= CRTSCTS; // Set baud rate to 19200 cfsetispeed(&tty, B19200); cfsetospeed(&tty, B19200); // Set 7 bits per byte tty.c_cflag |= CS7; // Enable even parity bit, without PARODD tty.c_cflag |= PARENB; // Set 2 stop bits tty.c_cflag |= CSTOPB; // Apply the new settings if (tcsetattr(fd, TCSANOW, &tty) < 0) { perror("Error setting port attributes"); close(fd); return -1; } printf("Serial port configured successfully\n"); printf("Port configuration:\n"); printf("- Device: %s\n", device); printf("- Baud rate: 19200\n"); printf("- Data bits: 7\n"); printf("- Parity: Even\n"); printf("- Stop bits: 2\n"); printf("- Flow control: RTS/CTS\n"); // Test string to send const char *test_string = "Hello! This is UART1\n"; ssize_t bytes_written = write(fd, test_string, strlen(test_string)); if (bytes_written < 0) { perror("Error writing to serial port"); } else { printf("\nSent %zd bytes: %s", bytes_written, test_string); } // Keep the port open briefly to ensure transmission sleep(1); // Close the serial port close(fd); return 0; }
-
回复: F133 RTSCTS LINE
check device tree first, check uart1_pins_a with RTS/CTS pin control
pinctrl-0 = <&uart1_pins_a>;
-
回复: T113-S3,adb shell乱码[T113_QA0002]
不要用Cmd 這沒法支持
用Putty Tray 試試
或是 PowerShell
另外還有Cmder
-
回复: V3s两个串口同时打开报错
@b18770205274
不是喔
你的語法是 send_fd > 0 就會執行 printf("open error\n"); exit(EXIT_FAILURE);要 if ((send_fd < 0) || (receive_fd < 0))這樣才是你說的意思
-
回复: H618怎么才能支持sdio 3.0模式
就dts 可能有問題
如果你都確定其他版本可以正確
表示在那個版本的軟硬件設定正確那事情就簡單了 就是Android 12 設定不正確
我也不是原廠的工程師
只是說明訊息產生的原因
言盡於此 -
回复: H618怎么才能支持sdio 3.0模式
@gwx123
電壓要能支持1.8V and 3.3V切換才行支持 SDIO 3.0
不然就吐那個說你的卡需要更低電壓 -
回复: A133芯片uboot运行时,SDK的uboot源码电源芯片有一个2s的超时机制,是否可将这个超时时间减少
沒試過不過看起來這段代碼有bugs
應該 if() else break; 讀到後就離開 while() -
回复: Ubuntu18.04配置livesuit环境时报错insmod: ERROR: could not insert module awusb.ko: Invalid module format
@ary_ye 在 Ubuntu18.04配置livesuit环境时报错insmod: ERROR: could not insert module awusb.ko: Invalid module format 中说:
linxiaoyan@WP:~/sunxi-livesuite-master/awusb$ modinfo ./awusb.ko filename: /home/linxiaoyan/sunxi-livesuite-master/awusb/./awusb.ko license: GPL description: AW USB driver author: Jojo srcversion: E085DDDF9EB284306C544FA alias: usb:v1F3ApEFE8d*dc*dsc*dp*ic*isc*ip*in* depends: retpoline: Y name: awusb vermagic: 5.15.0-41-generic SMP mod_unload modversions linxiaoyan@WP:~/sunxi-livesuite-master/awusb$ uname -r 5.15.0-41-generic linxiaoyan@WP:~/sunxi-livesuite-master/awusb$ sudo insmod ./awusb.ko [sudo] linxiaoyan 的密码: insmod: ERROR: could not insert module ./awusb.ko: Invalid module format linxiaoyan@WP:~/sunxi-livesuite-master/awusb$
内核版本对的上,livesuit上说重新安装dkms我也照做了,还是用不了,奇怪了
這樣我就也搞不懂了,我遇過的是版本對不上,livesuit 上的Makefile & dkms.conf 有問題
當系統kernel 升級的時候,它dkms 可能在前一個版本執行,uname -r 會有問題
要修改,我回答你問題的時候就發現我用5.15.0-116-generic 但是它會變成用5.15.0-113-generic
重新編譯才正常
你的系統有沒有開Secure Boot? 有的話只能用dkms 去編譯跟簽名
下面是我改過得 Makefile & dkms.conf
Makefilecode_text obj-m := awusb.o KDIR ?= /lib/modules/$(shell uname -r)/build PWD := $(shell pwd) default: $(MAKE) -C $(KDIR) M=$(PWD) modules clean: $(MAKE) -C $(KDIR) M=$(PWD) clean rm -rf Module.markers module.order module.sysvers
dkms.conf
code_text PACKAGE_NAME="awdev" PACKAGE_VERSION="0.5" CLEAN="make clean" MAKE[0]="make default KERNELVERSION=$kernelver KDIR=/lib/modules/$kernelver/build" BUILT_MODULE_NAME[0]="awusb" DEST_MODULE_LOCATION[0]="/updates" AUTOINSTALL="yes"
-
回复: Ubuntu18.04配置livesuit环境时报错insmod: ERROR: could not insert module awusb.ko: Invalid module format
@ary_ye
一般是linux kernel header 版本跟你正在使用的kernel 版本不同
用 modinfo 檢查編譯出來的 awusb.ko -
回复: 【T527 SDK编译报错】
我用Docker 裝 ubuntu 20.04
安裝下面 package 可以正常build core and full 版本
RUN dpkg --add-architecture i386 && \ apt-get update && \ apt-get install -y locales && \ localedef -i en_US -c -f UTF-8 -A /usr/share/locale/locale.alias en_US.UTF-8 ENV LANG en_US.UTF-8 RUN apt-get install --no-install-recommends --no-install-suggests --yes \ build-essential ca-certificates gcc gcc-multilib clang curl \ git git-lfs gnupg gperf build-essential zip libc6-dev python \ x11proto-core-dev libgl1-mesa-dev g++-multilib tofrodos zlib1g-dev \ libncurses5-dev:i386 libx11-dev:i386 libreadline6-dev:i386 \ libgl1-mesa-glx:i386 zlib1g-dev:i386 linux-libc-dev:i386 gawk \ libncurses5-dev bc bison flex gettext libssl-dev autoconf libtool \ wget patch dos2unix tree u-boot-tools libelf-dev libncurses5 \ libxml2-utils xsltproc markdown texinfo gettext busybox \ fakeroot cpio unzip rsync ccache xxd vim liblzo2-2 && \ apt-get clean && \ rm -rf /var/lib/apt/* /var/cache/apt/* /tmp/* /var/tmp/*
後面是完整的 Dockerfile 還有怎樣build docker image 跟進入docker環境用的shell script
進 container 後
直接 ./build.sh config && ./build.sh 即可
文件講的環境變數
只需要
export ARCH=arm64
export CROSS_COMPILE=aarch64-none-linux-gnu-
用我的Dockerfile 已經寫在裡面了
加上其他會產生類似你的錯誤
entrycontainer
build-container
Dockerfile -
回复: H616 需要 XPOWERS APX313A 吗?
基本上PMIC只是保證時序要求,你如果用獨立的DC/DC 可以完成時序要求沒有不行的
對H616本身來說它不關心外部器件實質為何,只關心是否吻合時序,電壓/電流等條件是否能滿足
當然如果你需要動態調整電壓功能的話那對應的DC/DC 也需要有對應的驅動程序 -
回复: 有没有搞过蓝牙wifi模块 RF测试的啊
@skrlou 在 有没有搞过蓝牙wifi模块 RF测试的啊 中说:
我是想问下有没有搞过这个的别的模块也可以 反正都是要厂商提供模块相对应的测试软件来搞 是想问下这个过程
就是搞過才跟你說就是按文件做
要看你的toolchain 编译出wifi_test,bt_test
然後 也要在你現在用的kernel 下編譯出文檔說的aic8800_fdrv.ko
看是廠商給你的東西怎樣去修改放到kernel 或是直接可以用ext modules
編譯的方法
ref https://docs.kernel.org/kbuild/modules.html
其他就是按文檔說給測試實驗室使用 -
回复: D1S 调试串口uart3,如何配置,需修改哪些文件
@westwhale
在
全志芯片Tina Linux 修改 UART 引脚、UART端口
https://bbs.aw-ol.com/topic/1673修改启动bootargs
有這個
你有做嗎?
這個決定linux 的console -
回复: 求高人指点! ISP通路测试 sample_vin_isp_test 报错
@xjy_5 如果你用原廠camera 那不關閉ISP的情況下會出錯嗎?
先找一個會動的案例出來
之後基於這個基礎去理解跟修改會不會好一點? -
回复: 求高人指点! ISP通路测试 sample_vin_isp_test 报错
@xjy_5
沒實際做過
不過看你的log 你可以看到是有呼叫到 驅動的但是 image pipeline 建立失敗
你仔細研究看看 應該有機會的I0101 03:45:59.705919 1128 alsa_interface.c:736] <alsaOpenMixer> alsa_elem:MIC2 In[ 32.135476] [tc358743_mipi]sensor_power put Select I0101 03:45:59.70604[ 32.142119] [tc358743_mipi]PWR_ON! 1 1128 alsa_interface.c:736] <alsaOpenMixer> alsa_elem:MIC2 gain volume I0101 03:45:59.706113 1128 alsa_interface.c:736] <alsaOpenMixer> alsa_elem:SPK I0101 03:45:59.706173 1128 alsa_interface.c:736] <alsaOpenMixer> alsa_elem:digital volume I0101 03:45:59.706228 1128 alsa_interface.c:736] <alsaOpenMixer> alsa_elem:rx sync mode I0101 03:45:59.706281 1128 alsa_interface.c:736] <alsaOpenMixer> alsa_elem:tx hub mode I0101 03:45:59.712543 1128 sample_virvi.c:633] <initSaveB[ 32.193321] [tc358743_mipi]sensor_init ufMgr> node[0] alloc data len[3110400] phy addr[1221591040] vir addr[0xb6966000] I0101 03:45:59.719106 1128 sample_virvi.c:633] <initSaveBufMgr> node[1] alloc data len[3110400] phy addr[1224736768] vir addr[0xb666e000] I0101 03:45:59.725542 1128 sample_virvi.c:633] <initSaveBufMgr> node[2] alloc data len[3110400] phy addr[1227882496] vir addr[0xb6376000] I0101 03:45:59.731885 1128 sample_virvi.c:633] <initSaveBufMgr> node[3] alloc data len[3110400] phy addr[1231028224] vir addr[0xb607e000] I0101 03:45:59.738354 1128 sample_virvi.c:633] <initSaveBufMgr> node[4] alloc data len[3110400] phy addr[1234173952] vir addr[0xb5d86000] [ISP]video device name is vin_video0 [ISP]open video device[0], detect isp0 success! I0101 03:45:59.739078 1128 media_debug.c:45] <getDictByConfPath> MPP_DEDIA_DEBUG_FILE_PATH=(null) I0101 03:45:59.739625 1128 videoInputHw.c:1015] <videoInputHw_SetChnAttr> ViCh[0], user set disable Encpp [ 32.375465] v4l2_dev:ddc438b8 [ 32.379612] [TC358743]tc358743 chipid:0x0 [ISP]video0 fd[14] ve_online_en=[ 32.384193] [VIN]ve_online close 0, dma_buf_num=0 [ 32.391026] [VIN_ERR]vin is not support this pixelformat [ 32.398110] [VIN_ERR]vin_pipeline_try_format failed [ISP_ERR]video_set_fmt, line: 194,VIDIOC_S_FMT error! E0101 03:[ 32.406800] [tc358743_mipi]sensor_ioctl cmd:-1069787396 46:00.008377 1128 videoInputHw.c:1055] <videoInputHw_SetChnAttr> video set_fmt failed, chn[0] E0101 03:46:00.008512 1128 sample_virvi.c:758] <prepare> fatal error! vi dev[0] set vipp attr fail! [ISP]open isp device[0] success! [ISP_ERR]isp_sensor_get_configs, line: 810,tc358743_mipi get config failed: Invalid argument (22). [ISP_WARN]open /mnt/extsd/isp0_0_0_0_ctx_saved.bin failed, err:No such file or directory. [ISP]prefer isp config: [tc358743_mipi], 0x0, 0, 0, 0 [ISP_WARN]cannot find tc358743_mipi_0_0_0_0_0 isp config, use gc2053_mipi_1920_1088_20_0_0 -> [gc2053_mipi_isp600_20220511_164617_vlc4_day] [ISP_ERR]isp_ctx_config_init, line: 2305,sensor attribute is not init. [ISP]create isp0 server thread! planes number is error!
記得你的 ISP 要trun off
ref.https://zhuanlan.zhihu.com/p/628496408 -
回复: 有人尝试把Docker的代码拷贝到本地进行编译吗
@mysteryli
check the https://docs.docker.com/engine/install/linux-postinstall/
for non-root user with docker -
回复: 有关编译c内联矢量指令时,unknown register name ‘v1’ in ‘asm’的问题
@zny666
Which filesystem fat or ext4 on SDCARD?
maybe try copy ./tmp3 on ext4 filesystem to verify again,cp -vf tmp3 /tmp && chmod a+x /tmp/tmp3 && /tmp/tmp3
-
回复: 有关编译c内联矢量指令时,unknown register name ‘v1’ in ‘asm’的问题
@zny666
first try
chmod a+x ./tmp3
then run
sudo ./tmp3 -
如何用 LiveSuite 刷固件?
想请教一下LiveSuite 现在在Linux 上面刷固件有啥要注意的没有
不知道为啥进了FEL mode 后, LiveSuite 只会提示是否要格式化 然后就没反应了
用dmesg 會出現 task timeout 如下面兩張圖
task timeout
-
回复: 芒果派t113s3板子的tina,ubuntu用哪个版本比较好?22.04似乎不少问题
@newcastle
我自己测试 在目前Allwinner 系列上面 Ubuntu 18.04 问题最少 -
回复: 请问大佬怎么转换FEX文件 内有图片
@lknn2012
看你的2.
simg2imag system.fex 应该是android spare to raw 就变成 ext4
应该可以用img2simg 还原才对
看看这个
https://blog.csdn.net/jingwen3699/article/details/81807024 -
使用Docker 编译 Tina-Linux
Docker image 使用Ubuntu 18.04 当基底
目前验证D1-H NeZha Tina-Linux v2.x 编译正常
原则上应该也能编译A133
git config 要在主机 环境先设定
build docker image 会直接使用它Dockerfile 內容
FROM ubuntu:18.04 ARG userid ARG groupid ARG username RUN apt-get update && apt-get install -y \ build-essential cvs subversion mercurial git-core \ libncurses5-dev zlib1g-dev gawk flex quilt openssl libssl-dev \ xsltproc libxml-parser-perl bzr ecj unzip \ lib32z1 lib32z1-dev libc6-i386 lib32ncurses5 \ lib32stdc++6 libstdc++6 rsync vim wget curl bc busybox \ linux-tools-common gperf && \ apt-get clean && \ rm -rf /var/lib/apt/lists/* /tmp/* /var/tmp/* RUN curl -o /usr/local/bin/repo https://storage.googleapis.com/git-repo-downloads/repo \ && chmod a+x /usr/local/bin/repo RUN groupadd -g $groupid $username \ && useradd -m -u $userid -g $groupid $username \ && echo $username >/root/username \ && echo "export USER="$username >>/home/$username/.gitconfig COPY gitconfig /home/$username/.gitconfig RUN chown $userid:$groupid /home/$username/.gitconfig ENV HOME=/home/$username ENV USER=$username ENV WORKFOLDER=/home/$username ENTRYPOINT chroot --userspec=$(cat /root/username):$(cat /root/username) / /bin/bash -c "cd $WORKFOLDER && exec /bin/bash -i"
编译这个Dockerfile 我使用下面指令
#!/bin/bash cp ~/.gitconfig ./gitconfig docker build --build-arg userid=$(id -u) --build-arg groupid=$(id -g) --build-arg username=$(id -un) --tag allwinner-tina:latest .
然后在下载好的源代码目录建立一个执行的 script 叫做 entrycontainer.sh
内容如下#!/bin/bash WORKSPACE=$PWD docker run --privileged \ --interactive \ --tty \ --rm \ --volume=/tmp:/tmp \ --volume=${WORKSPACE}:/tina \ --env WORKFOLDER=/tina \ --hostname tina-build \ allwinner-tina:latest
使用方式如下图
-
有A133 的 GMAC 跟RTL8363NB 的原理图?
有没有人有 A133 的 GMAC 跟RTL8363NB 之间的原理图
目前看 A133/R818 一般接 RTL8112F,
RTL8112F 有输出125MHz给 A133 PH3/RGM0_CLKIN
但是RTL8363NB 没有这个125MHz 只有RX/TX Clock 这样可以用吗?